How to Stop a Transcode Session Automatically
Let me start by saying that this is one of those "just because you could, doesn't mean you should" posts. I say that because I wouldn't advise you to run a script on your server that automatically stops transcode sessions. It isn't something your users would enjoy.
Then again, it is your server, and you can do as you please.
Below I present two small Python scripts that can be used to automatically stop any transcode session that starts on your server, and one script allows you to send a message to your users.
Terminate a transcode session
The first script I will provide will call two Plex API commands. The first one is the Listen for Events command that will establish a connection to your Plex server, and Plex will send notifications when certain events occur.
The second is the Terminate a Transcode Session command that will stop a transcode session.
The script to terminate a transcode session automatically on a Plex server is shown below:
from sseclient import SSEClient import os import json import requests # Get the Plex URL and token from the environment variables plex_url = os.environ.get('PLEX_URL') plex_token = os.environ.get('PLEX_TOKEN') # Establish a connection to the Plex server to have Plex send notifications messages = SSEClient('{0}/:/eventsource/notifications?X-Plex-Token={1}'.format(plex_url, plex_token)) for msg in messages: data = json.loads(msg.data) # Look for a TranscodeSession message that indicates a transcode is being done if 'TranscodeSession' in data: if data['TranscodeSession']: # Get the transcode session key and use that to create the terminate # transcode session API command session_id = data['TranscodeSession']["key"] url = '{0}{1}?X-Plex-Token={2}'.format(plex_url, session_id, plex_token) requests.delete(url)
I have added comments to the code, so I won't go through it line-by-line. It establishes a connection to the Plex server and waits to receive notifications. When a TranscodeSession
message is received, the session key is stored and then used to terminate the session.
The issue with using this script is that the user will get a message on their screen that isn't very descriptive.
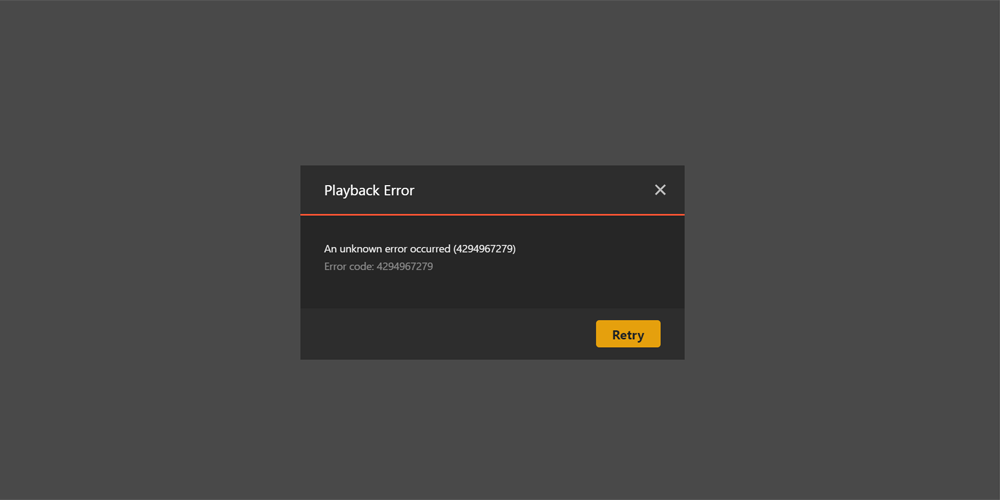
When a user sees this message there could be more "support" calls to you regarding playback on your server. The next script will allow you to display a more meaningful message to your users.
Terminate a transcode session with a message
If you are going to automatically stop transcoding from happening on your server, the best option would be to tell your users why their playback ended.
The script to do that is a little more complex as we will need to make additional API calls to the Plex server.
The script will make the same call to listen for events from the Plex server, but instead of terminating a transcode session directly, it will terminate the entire session.
This is done by calling the Get Active Sessions API command to find the session for the transcode, and then calling the Terminate a Session command to stop the session and provide a message to the user.
The script to accomplish this is below:
from sseclient import SSEClient import os import json import urllib import requests import xml.etree.ElementTree as ET # Get the Plex URL and token from the environment variables plex_url = os.environ.get('PLEX_URL') plex_token = os.environ.get('PLEX_TOKEN') # Establish a connection to the Plex server to have Plex send notifications messages = SSEClient('{0}/:/eventsource/notifications?X-Plex-Token={1}'.format(plex_url, plex_token)) for msg in messages: data = json.loads(msg.data) # Look for a TranscodeSession message that indicates a transcode is being done if 'TranscodeSession' in data: if data['TranscodeSession']: # Get the transcode session key and store the active session API URL session_id = data['TranscodeSession']["key"] sessions_url = '{0}/status/sessions/?X-Plex-Token={1}'.format(plex_url, plex_token) # Send an API request to the Plex server to get all active sessions # to find the session associated with the transcode session sent # in the message from Plex response = requests.get(sessions_url) if response.ok: root = ET.fromstring(response.content) # Loop through all the active video sessions as those are the # session we are looking for for video_tag in root.findall('Video'): # Check if the video session contains a transcode session as # only those session will contain the TranscodeSession tag transcode_session_tag = video_tag.find('TranscodeSession') if transcode_session_tag is None: continue # Get the transcode key from the active session API command # and compare it with the key from the message key = transcode_session_tag.get('key') if key == session_id: session_tag = video_tag.find('Session') if session_tag is None: continue # Get the ID of the session, create a message to the user, # and then call the terminate session API using the session # ID returned by the active sessions API id = session_tag.get('id') reason = urllib.parse.quote_plus('This play session is terminating. Please adjust your quality settings.') url = '{0}/status/sessions/terminate?X-Plex-Token={1}&sessionId={2}&reason={3}'.format(plex_url, plex_token, id, reason) requests.get(url)
Once again I provided comments in the code to help understand what the script is doing. The end of the script shows the call to stop the session and provides a reason for stopping the session.
The above script will produce a message similar to the following:
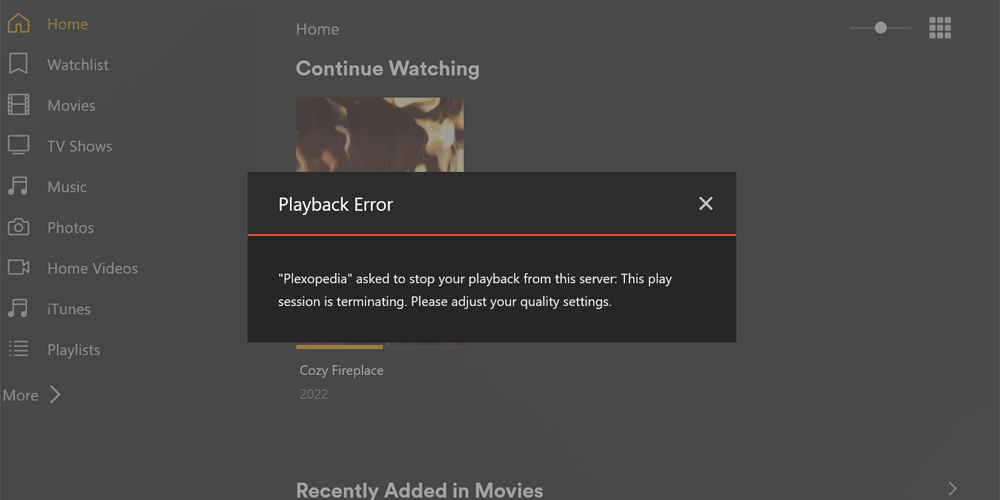
This message is a bit more helpful to the user, and you can be more detailed in what you display. Perhaps providing information on how they can change the quality settings in their Plex client would be helpful.
Client information returned by the active sessions API call also returns information about the player used to stream the media. Maybe use that to provide a more specific message to the user about adjusting their quality settings.
Instead of running scripts, you can just disable video transcoding in the Plex settings to achieve something similar.
How to use the scripts
To use the script, you will need to do the following:
- Install Python.
- After Python is installed, run the following
pip
command to install the dependencies:pip install requests pip install urllib3 pip install sseclient
- Copy either one or both of the above scripts and save each as a Python file, for example:
terminate_transcode_1.py
andterminate_transcode_2.py
- Create an environment variable called
PLEX_URL
and set it to the URL of your Plex server. For example:http://localhost:32400
. - Create an environment variable called
PLEX_TOKEN
and set it to your Plex token.
While I have made the above two scripts available in this post I don't run them on my Plex server, and never will.
There are additional steps you can take to avoid transcoding on your server, and these steps are what I prefer to do.